Components
3D Cards
Interactive 3D cards with realistic depth effects powered by Tailwind CSS 4. These cards respond to mouse movement, creating an immersive user experience with customizable depth, perspective, and glare effects.
Senior
Development Team
Alex Johnson
Full Stack Developer with 8+ years of experience specializing in React, Node.js, and cloud architecture.
56
Projects
8+
Years Exp.
12
Awards
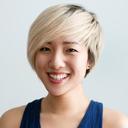

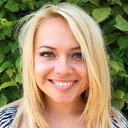
Team Collaboration
Frontend Squad
Component Documentation
Prop | Type | Default Value | Description |
---|---|---|---|
children | string | Card content | |
className | string | "" | Additional CSS classes |
width | string | "w-full md:w-80" | Card width (Tailwind classes) |
height | string | "h-auto" | Card height (Tailwind classes) |
depth | string | "depth-10" | 3D depth effect (Tailwind CSS 4 utility) |
perspective | string | "perspective-1000" | Perspective value for 3D effect |
rotateAmount | string | 15 | Maximum rotation angle in degrees |
scaleOnHover | string | 1.05 | Scale factor on hover |
bgColor | string | "bg-white dark:bg-gray-800" | Background color (dark mode compatible) |
textColor | string | "text-gray-900 dark:text-gray-100" | Text color (dark mode compatible) |
borderColor | string | "border-gray-200 dark:border-gray-700" | Border color (dark mode compatible) |
shadowColor | string | "shadow-black/5 dark:shadow-black/30" | Shadow color (dark mode compatible) |
rounded | string | "rounded-xl" | Border radius |
padding | string | "p-6" | Internal padding |
border | string | "border" | Border style |
shadow | string | "shadow-lg" | Shadow style |
transition | string | "transition-transform duration-300 ease-out" | Transition effect |
interactive | string | true | Whether the card responds to mouse movement |
glare | string | false | Whether to show a glare effect |
glareOpacity | string | 0.1 | Opacity of the glare effect (0-1) |
glarePosition | string | {"x":0,"y":0} | Initial position of the glare effect |
onClick | string | function() | Click event handler |
Usage Example
Usage Example
jsx
"use client";
import React from "react";
import Card3D from "@/tailwind/foraui/components/3d/Card";
import FloatingBadge from "@/tailwind/foraui/components/badges/FloatingBadge";
/**
* Demo component showcasing a single elegant 3D card for team member presentation
* Designed to be used within a Showcase component
*/
const Card3DDemo = () => {
return (
<div className="w-full flex items-center justify-center py-2 sm:py-4 max-w-full">
<Card3D
width="w-full max-w-[280px] sm:max-w-[320px] md:max-w-sm"
height="h-auto"
depth="depth-10 sm:depth-20"
perspective="perspective-1000 sm:perspective-1500"
rotateAmount={8}
scaleOnHover={1.02}
glare={true}
glareOpacity={0.12}
bgColor="bg-gradient-to-br from-white to-gray-50 dark:from-gray-800 dark:to-gray-900"
borderColor="border-gray-100 dark:border-gray-700"
shadow="shadow-lg sm:shadow-xl"
shadowColor="shadow-blue-500/10 dark:shadow-blue-500/20"
rounded="rounded-xl sm:rounded-2xl"
padding="p-0"
className="overflow-hidden"
>
<div className="relative">
{/* Profile Image */}
<div className="h-32 sm:h-40 overflow-hidden">
<img
src="https://images.unsplash.com/photo-1568602471122-7832951cc4c5?ixlib=rb-4.0.3&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=2070&q=80"
alt="Team Member Profile"
className="w-full h-full object-cover"
/>
{/* Floating badge */}
<div className="absolute top-3 right-3 sm:top-4 sm:right-4">
<FloatingBadge label="Senior" />
</div>
</div>
{/* Content */}
<div className="p-3 sm:p-4 md:p-5">
<div className="flex items-center gap-2 mb-2 sm:mb-3">
<div className="h-px flex-1 bg-gradient-to-r from-transparent via-gray-200 dark:via-gray-700 to-transparent"></div>
<span className="text-[10px] sm:text-xs font-medium text-gray-500 dark:text-gray-400 uppercase tracking-wider">
Development Team
</span>
<div className="h-px flex-1 bg-gradient-to-r from-transparent via-gray-200 dark:via-gray-700 to-transparent"></div>
</div>
<h2 className="text-lg sm:text-xl font-bold mb-1.5 sm:mb-2 text-gray-900 dark:text-white">
Alex Johnson
</h2>
<p className="text-xs sm:text-sm text-gray-600 dark:text-gray-300 mb-3 sm:mb-4">
Full Stack Developer with 8+ years of experience specializing in
React, Node.js, and cloud architecture.
</p>
{/* Stats */}
<div className="grid grid-cols-3 gap-1 sm:gap-2 mb-3 sm:mb-4">
<div className="text-center">
<div className="text-base sm:text-lg font-bold text-primary-600 dark:text-primary-400">
56
</div>
<div className="text-[10px] sm:text-xs text-gray-500 dark:text-gray-400">
Projects
</div>
</div>
<div className="text-center">
<div className="text-base sm:text-lg font-bold text-primary-600 dark:text-primary-400">
8+
</div>
<div className="text-[10px] sm:text-xs text-gray-500 dark:text-gray-400">
Years Exp.
</div>
</div>
<div className="text-center">
<div className="text-base sm:text-lg font-bold text-primary-600 dark:text-primary-400">
12
</div>
<div className="text-[10px] sm:text-xs text-gray-500 dark:text-gray-400">
Awards
</div>
</div>
</div>
{/* Action buttons */}
<div className="flex gap-1.5 sm:gap-2">
<button className="flex-1 px-2 sm:px-3 py-1.5 sm:py-2 bg-primary-600 hover:bg-primary-700 text-white rounded-md sm:rounded-lg transition-colors text-[10px] sm:text-xs font-medium">
Contact
</button>
<button className="px-2 sm:px-3 py-1.5 sm:py-2 border border-gray-200 dark:border-gray-700 hover:bg-gray-50 dark:hover:bg-gray-800 text-gray-700 dark:text-gray-300 rounded-md sm:rounded-lg transition-colors text-[10px] sm:text-xs font-medium">
View Profile
</button>
</div>
</div>
{/* Footer */}
<div className="px-3 sm:px-4 md:px-5 py-2 sm:py-3 border-t border-gray-100 dark:border-gray-800 bg-gray-50/50 dark:bg-gray-900/50">
<div className="flex items-center justify-between">
<div className="flex items-center gap-1.5 sm:gap-2">
<div className="flex -space-x-1.5">
<img
src="https://randomuser.me/api/portraits/women/44.jpg"
alt="Team Member"
className="w-5 h-5 sm:w-6 sm:h-6 rounded-full border-2 border-white dark:border-gray-800"
/>
<img
src="https://randomuser.me/api/portraits/men/32.jpg"
alt="Team Member"
className="w-5 h-5 sm:w-6 sm:h-6 rounded-full border-2 border-white dark:border-gray-800"
/>
<img
src="https://randomuser.me/api/portraits/women/68.jpg"
alt="Team Member"
className="w-5 h-5 sm:w-6 sm:h-6 rounded-full border-2 border-white dark:border-gray-800"
/>
</div>
<div>
<div className="text-[10px] sm:text-xs font-medium text-gray-900 dark:text-white">
Team Collaboration
</div>
<div className="text-[8px] sm:text-xs text-gray-500 dark:text-gray-400">
Frontend Squad
</div>
</div>
</div>
<div className="flex gap-0.5 sm:gap-1">
<button className="p-0.5 sm:p-1 rounded-full text-gray-500 hover:text-gray-700 dark:text-gray-400 dark:hover:text-gray-200 hover:bg-gray-100 dark:hover:bg-gray-800 transition-colors">
<svg
xmlns="http://www.w3.org/2000/svg"
className="h-3 w-3 sm:h-3.5 sm:w-3.5"
viewBox="0 0 20 20"
fill="currentColor"
>
<path d="M15 8a3 3 0 10-2.977-2.63l-4.94 2.47a3 3 0 100 4.319l4.94 2.47a3 3 0 10.895-1.789l-4.94-2.47a3.027 3.027 0 000-.74l4.94-2.47C13.456 7.68 14.19 8 15 8z" />
</svg>
</button>
<button className="p-0.5 sm:p-1 rounded-full text-gray-500 hover:text-gray-700 dark:text-gray-400 dark:hover:text-gray-200 hover:bg-gray-100 dark:hover:bg-gray-800 transition-colors">
<svg
xmlns="http://www.w3.org/2000/svg"
className="h-3 w-3 sm:h-3.5 sm:w-3.5"
viewBox="0 0 20 20"
fill="currentColor"
>
<path
fillRule="evenodd"
d="M3.172 5.172a4 4 0 015.656 0L10 6.343l1.172-1.171a4 4 0 115.656 5.656L10 17.657l-6.828-6.829a4 4 0 010-5.656z"
clipRule="evenodd"
/>
</svg>
</button>
<button className="p-0.5 sm:p-1 rounded-full text-gray-500 hover:text-gray-700 dark:text-gray-400 dark:hover:text-gray-200 hover:bg-gray-100 dark:hover:bg-gray-800 transition-colors">
<svg
xmlns="http://www.w3.org/2000/svg"
className="h-3 w-3 sm:h-3.5 sm:w-3.5"
viewBox="0 0 20 20"
fill="currentColor"
>
<path d="M6 10a2 2 0 11-4 0 2 2 0 014 0zM12 10a2 2 0 11-4 0 2 2 0 014 0zM16 12a2 2 0 100-4 2 2 0 000 4z" />
</svg>
</button>
</div>
</div>
</div>
</div>
</Card3D>
</div>
);
};
export default Card3DDemo;